Pre-processing example (with HYDROLIB-core)#
[1]:
#NOTE: this notebook does not run in binder, since the data is not yet available online
import os
import numpy as np
import matplotlib.pyplot as plt
plt.close('all')
import dfm_tools as dfmt
import hydrolib.core.dflowfm as hcdfm
[2]:
#Load .bc-file using HydroLib object ForcingModel.
file_bc = r'p:\archivedprojects\11208053-004-kpp2022-rmm1d2d\C_Work\09_Validatie2018_2020\dflowfm2d-rmm_vzm-j19_6-v2d\boundary_conditions\rmm_rivdis_meas_20171101_20210102_MET.bc'
forcingmodel_object = hcdfm.ForcingModel(file_bc)
fig, ax = plt.subplots()
# loop over three timeseries in bcfile/ForingModel
for iFO, forcingobj in enumerate(forcingmodel_object.forcing):
forcing_xr = dfmt.forcinglike_to_Dataset(forcingobj, convertnan=True)
forcing_xr['dischargebnd'].plot(ax=ax, label=forcing_xr['dischargebnd'].attrs['locationname'], linewidth=0.8)
ax.legend(loc=1)
[2]:
<matplotlib.legend.Legend at 0x2a5d8d73950>
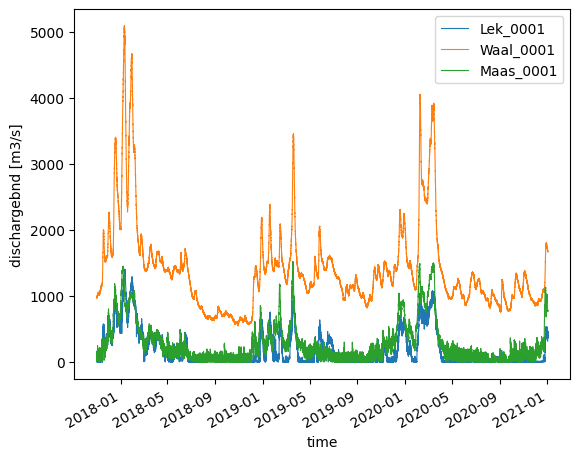
[3]:
#Load .bc-file using HydroLib object ForcingModel.
file_bc = r'p:\archivedprojects\11208154-002-haixia\02-hydrodynamics\02_Model_set_up\02_Make_forcing\FES2014\New_bnd_lines_2022\bc_South_v2.bc'
forcingmodel_object = hcdfm.ForcingModel(file_bc)
fig, ax = plt.subplots(figsize=(10,4))
ax2 = ax.twinx()
forcing_xr = dfmt.forcinglike_to_Dataset(forcingmodel_object.forcing[0], convertnan=True)
forcing_xr['waterlevelbnd amplitude'].plot(ax=ax, linewidth=0.8)
forcing_xr['waterlevelbnd phase'].plot(ax=ax2, linewidth=0.8)
[3]:
[<matplotlib.lines.Line2D at 0x2a5ccf97c90>]
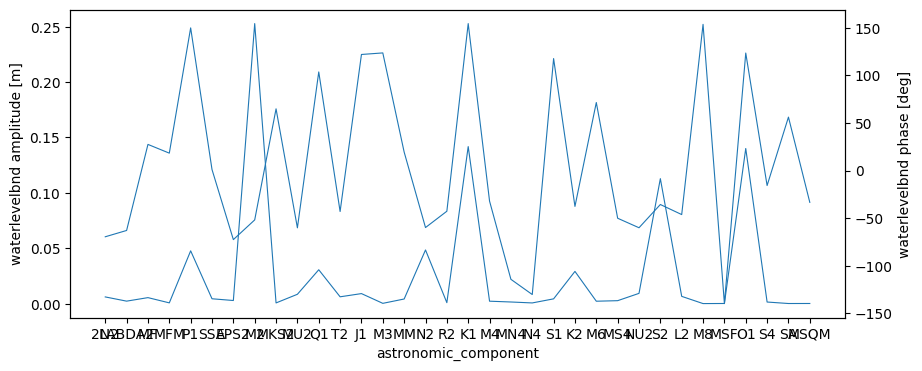
[4]:
#Load .bc-file using HydroLib object ForcingModel.
file_bc = r'p:\archivedprojects\11208154-002-haixia\02-hydrodynamics\02_Model_set_up\02_Make_forcing\CMEMS\bc_2022\South_v2\nonan\salinitybnd_bc_South_v2_CMEMS.bc'
forcingmodel_object = hcdfm.ForcingModel(file_bc)
fig, ax = plt.subplots()
forcing_xr = dfmt.forcinglike_to_Dataset(forcingmodel_object.forcing[0], convertnan=True)
forcing_xr['salinitybnd'].T.plot(ax=ax)
[4]:
<matplotlib.collections.QuadMesh at 0x2a5cccea9d0>
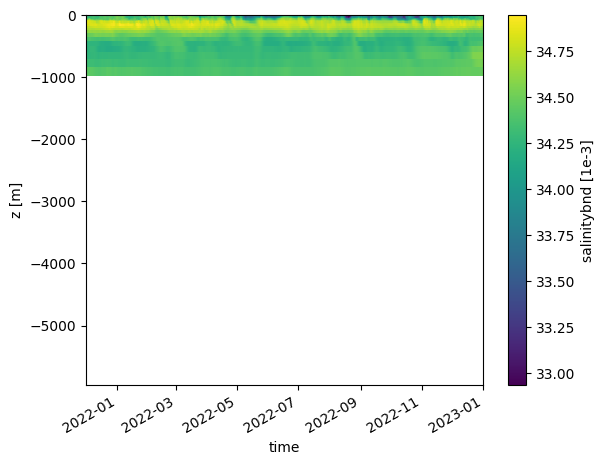
[5]:
#Load .bc-file using HydroLib object ForcingModel.
file_bc = r'p:\archivedprojects\11208154-002-haixia\02-hydrodynamics\02_Model_set_up\02_Make_forcing\CMEMS\bc_2022\South_v2\nonan\uxuy_bc_South_v2_CMEMS.bc'
forcingmodel_object = hcdfm.ForcingModel(file_bc)
fig, (ax1,ax2) = plt.subplots(2,1,sharex=True,sharey=True)
forcing_xr = dfmt.forcinglike_to_Dataset(forcingmodel_object.forcing[0], convertnan=True)
forcing_xr['ux'].T.plot(ax=ax1)
forcing_xr['uy'].T.plot(ax=ax2)
[5]:
<matplotlib.collections.QuadMesh at 0x2a5d2183950>
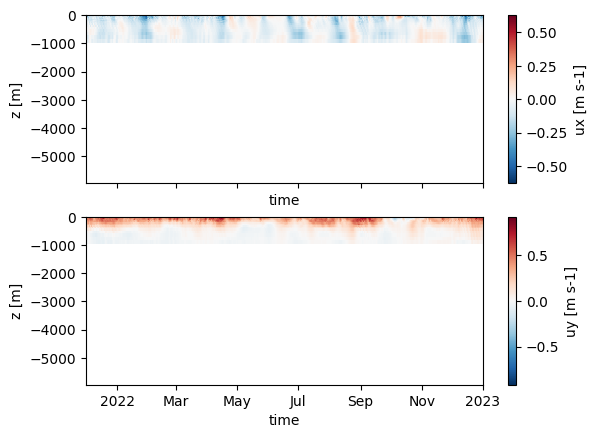
[6]:
#load pol/pli/ldb file WITH HYDROLIB-core
file_pli = r'p:\archivedprojects\11205259-006-d-hydro-grevelingen\2Dh\model\2002\geometry\structures\Grevelingen-FM_BL_fxw.pliz'
polyfile_object = hcdfm.PolyFile(file_pli)
gdf_polyfile = dfmt.PolyFile_to_geodataframe_linestrings(polyfile_object,crs='EPSG:28992')
ax = gdf_polyfile.plot()
ax.set_aspect('equal')
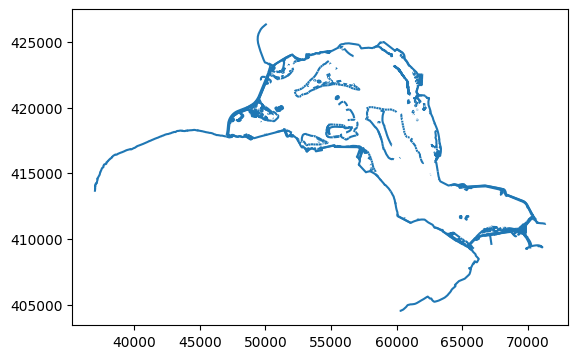
[7]:
#load tekal file WITH HYDROLIB-core
file_pli = r'p:\archivedprojects\11205258-006-kpp2020_rmm-g6\C_Work\04_randvoorwaarden\keringen\Maeslantkering\Maeslant.tek'
polyfile_object = hcdfm.PolyFile(file_pli)
polyobject_pd = dfmt.tekalobject_to_DataFrame(polyfile_object.objects[0])
ax = polyobject_pd.plot()
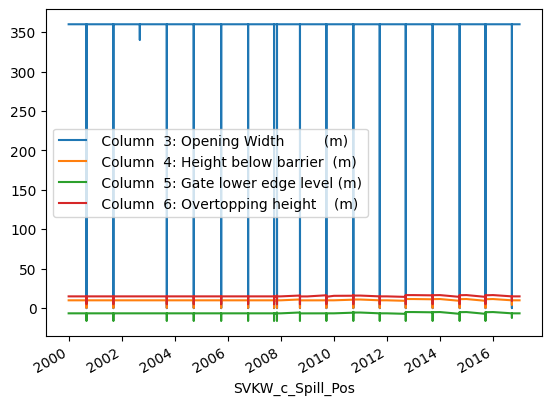
[ ]: